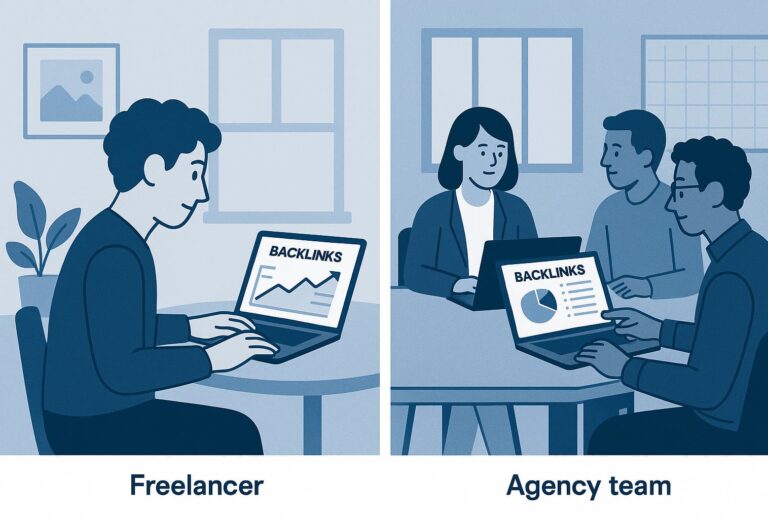
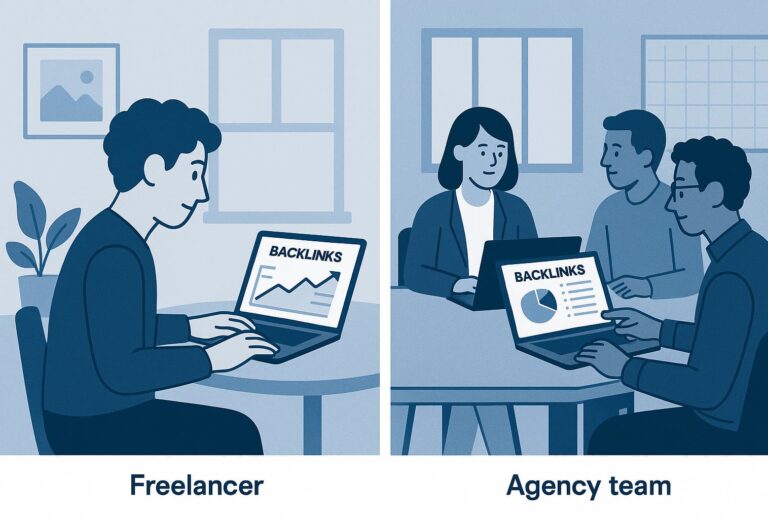
Open code – open possibilities!
Everything you need to know about the world of JavaScript!
At ID Flat Roof, we’re the roofer you can count on for long-lasting PVC flat roofing solutions. Whether it’s a residential or commercial project, our skilled team delivers top-tier results every time. Check out our gallery and customer reviews - then reach out for expert guidance and a personalized consultation.
Modern JS technologies are actively developing new online slots, offering interesting interactive graphics and effects. Play the best games at GG.Bet casino, test strategies and win in the proven newest slots in the iGaming industry.
Theory-Talks.org helps UK players discover and compare non Gamstop casinos. It provides clear and honest reviews of offshore sites. These casinos don’t follow the Gamstop rules and give players more freedom and convenience.
Lucky-7-Bonus.fr is the #1 site for discovering and comparing the best online casinos. Get an exclusive bonus and free spins on your favorite slot machine right now.
A casino not on GamStop gives you more control — skip verification, use Bitcoin, and access exclusive offers without UK restrictions. Find out where to play now.
Discover the best casinos not on GamStop offering crypto deposits, no UK self-exclusion, and big welcome deals. Ideal for players who want freedom and fast withdrawals.
Get access to advanced trading features through minereum.com, including MetaTrader 4 & 5 for forex, CFD, and crypto markets.
Ensure that your initial deposit is multiplied by five! With this incredible 400% match bonus, you may indulge in additional slot machines, table games, and live casino action.
Curious about how no deposit bonus codes work? Learn what these codes are, how to take advantage of them, and where to find them for gambling.