Integrating WebSockets in JavaScript for live market feeds allows developers to provide real-time data to users, creating a more interactive and engaging experience. Unlike traditional HTTP requests, WebSockets establish a persistent, full-duplex connection between the client and server, enabling continuous data flow. This is especially useful for applications that require frequent updates, such as financial platforms where users need up-to-the-second market data. By using WebSockets, applications can deliver low-latency updates, reducing the need for frequent polling and enhancing performance. In JavaScript, the WebSocket API facilitates the connection to WebSocket servers, enabling seamless integration of live market feeds directly into websites or apps.
One of the most effective applications of WebSockets in financial services is in trading platforms. For example, the Exness web terminal uses WebSockets to stream live market data, such as forex prices, stock quotes, and cryptocurrency values. By integrating WebSockets, the terminal can push real-time updates to traders, ensuring they never miss crucial market movements. This real-time data streaming empowers users to make informed decisions quickly, improving trading strategies. Developers can leverage WebSockets in their own applications to replicate this experience, offering users a dynamic, responsive interface for market interaction.
What Are WebSockets?
WebSockets are a communication protocol that provides full-duplex, persistent communication channels between a client (typically a web browser) and a server. Unlike traditional HTTP, where the client makes a request and the server responds, WebSockets allow for continuous, two-way communication, meaning both the client and server can send data to each other at any time. This is particularly beneficial for real-time applications, such as live chat, online gaming, financial trading platforms, and social media feeds, where frequent updates are necessary.
The WebSocket connection is initiated through an HTTP handshake, but once established, it upgrades to a WebSocket protocol, which remains open until either the client or the server closes it. This persistent connection eliminates the need for multiple requests, reducing the overhead and latency typically associated with repeated HTTP requests. WebSockets are especially powerful when dealing with real-time data, as they provide lower latency and more efficient bandwidth usage compared to traditional methods like polling or long-polling.
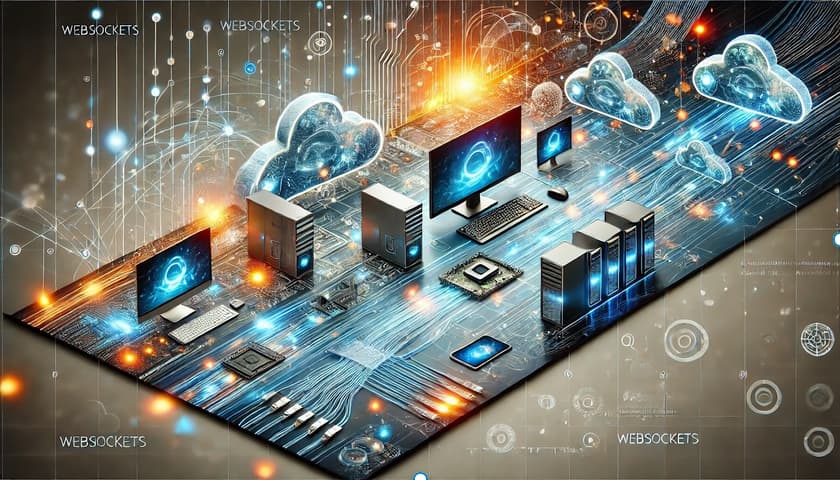
Setting Up WebSocket Server
Setting up a WebSocket server involves creating a backend that listens for WebSocket connections from clients and handles the communication between them. One of the most common ways to set up a WebSocket server is by using Node.js, as it provides an easy-to-use event-driven model that works well with WebSockets. Here’s a step-by-step guide to setting up a WebSocket server using Node.js and the popular ws library.
1. Install Node.js and ws Library
First, ensure you have Node.js installed. If not, download it from nodejs.org. Once Node.js is installed, you can install the ws library, which provides WebSocket functionality.
In your terminal, run:
npm init -y
npm install ws
This will initialize a new Node.js project and install the ws WebSocket library.
2. Create the WebSocket Server
Next, create a JavaScript file (e.g., server.js) and import the ws library to create a WebSocket server.
const WebSocket = require(‘ws’); // Import the WebSocket library
// Create a WebSocket server on port 8080
const wss = new WebSocket.Server({ port: 8080 });
// Handle new WebSocket connections
wss.on(‘connection’, ws => {
console.log(‘A new client has connected!’);
// Send a welcome message to the client
ws.send(‘Welcome to the WebSocket server!’);
// Listen for messages from the client
ws.on(‘message’, message => {
console.log(‘Received: ‘ + message);
});
// Handle the closing of the WebSocket connection
ws.on(‘close’, () => {
console.log(‘Client disconnected’);
});
});
console.log(‘WebSocket server is running on ws://localhost:8080’);
3. Running the Server
To start your WebSocket server, simply run the following command in your terminal:
node server.js
The server will start and listen for WebSocket connections on port 8080. You’ll see a message in the console confirming that the WebSocket server is running.
4. Connecting Clients to the WebSocket Server
Once the server is set up, clients can connect to it using the WebSocket protocol. Here’s an example of how you can connect to the WebSocket server from a browser using JavaScript.
const socket = new WebSocket(‘ws://localhost:8080’); // Connect to the WebSocket server
// Log a message when the connection is established
socket.onopen = () => {
console.log(‘Connected to the server’);
socket.send(‘Hello, server!’); // Send a message to the server
};
// Log any messages received from the server
socket.onmessage = (event) => {
console.log(‘Message from server: ‘ + event.data);
};
// Log any errors
socket.onerror = (error) => {
console.log(‘WebSocket Error: ‘ + error);
};
// Log when the connection is closed
socket.onclose = () => {
console.log(‘Disconnected from server’);
};
This code will establish a WebSocket connection from a web browser to the Node.js WebSocket server, send a message, and display any received messages.
5. Testing and Debugging
Once both the server and client are running, you can test the connection by opening the client in your browser and observing the interaction between the client and server. Make sure to check the console for any messages indicating successful connections or errors.
How WebSockets Integrating in JavaScript
Integrating WebSockets in JavaScript involves using the WebSocket API to establish a two-way communication channel between a client (usually a browser) and a server. The WebSocket protocol provides an efficient and low-latency way to push data to the client in real-time, making it ideal for applications like live market feeds, chat systems, multiplayer games, and more.
Here’s an overview of how to integrate WebSockets into a JavaScript application:
1. Creating a WebSocket Connection
To integrate WebSockets in JavaScript, the first step is to create a WebSocket object on the client side. You need to specify the URL of the WebSocket server to establish a connection. The URL will use either ws:// (for non-secure) or wss:// (for secure connections) depending on whether the server supports encrypted communication.
// Create a WebSocket connection to the server
const socket = new WebSocket(‘ws://localhost:8080’); // Change the URL to your WebSocket server
2. Handling WebSocket Events
WebSockets in JavaScript use events to communicate between the client and the server. The four most common events are:
- onopen: Triggered when the connection is successfully established.
- onmessage: Triggered when the server sends data to the client.
- onclose: Triggered when the WebSocket connection is closed.
- onerror: Triggered when there is an error with the WebSocket connection.
Each of these events is handled by defining corresponding functions in your JavaScript code.
Example: Listening for WebSocket Events
// When the WebSocket connection is opened
socket.onopen = () => {
console.log(‘WebSocket connection established!’);
socket.send(‘Hello, server!’); // Send a message to the server
};
// When a message is received from the server
socket.onmessage = (event) => {
console.log(‘Message from server:’, event.data); // Log the message
// You can process the message here (e.g., update the UI with new data)
};
// When the WebSocket connection is closed
socket.onclose = () => {
console.log(‘WebSocket connection closed’);
};
// When an error occurs with the WebSocket connection
socket.onerror = (error) => {
console.log(‘WebSocket Error:’, error);
};
3. Sending Data to the WebSocket Server
Once the connection is established, you can send data to the WebSocket server using the send() method. You can send strings, JSON data, or even binary data, depending on your application’s needs.
For example, to send a string:
socket.send(‘This is a message to the server’);
To send a JSON object, you should serialize it into a string:
javascript
КопіюватиРедагувати
const data = { type: ‘update’, message: ‘This is a market update’ };
socket.send(JSON.stringify(data)); // Send JSON as a string
4. Closing the WebSocket Connection
To gracefully close the WebSocket connection, you can use the close() method. This can be triggered either by the client or the server.
socket.close(); // Close the WebSocket connection
You can also provide a status code and a reason for closing the connection:
socket.close(1000, ‘Normal closure’); // 1000 is the normal closure code
5. Reconnecting WebSocket (Optional)
In real-world applications, WebSocket connections may be lost due to network issues or server downtimes. If maintaining a persistent connection is crucial, you may want to implement automatic reconnection logic. This can be done by listening for the onclose event and reopening the connection after a delay.
Example:
socket.onclose = () => {
console.log(‘Connection closed, attempting to reconnect…’);
setTimeout(() => {
socket = new WebSocket(‘ws://localhost:8080’); // Recreate the WebSocket connection
}, 1000); // Attempt to reconnect after 1 second
};
6. Handling Real-Time Market Feeds
When integrating WebSockets for live market feeds (e.g., stock prices, cryptocurrency updates, etc.), the server typically pushes market data to the client in real-time. In the client-side JavaScript, you’ll need to parse the incoming data and update the user interface dynamically.
For example:
socket.onmessage = (event) => {
const marketData = JSON.parse(event.data); // Assuming the data is in JSON format
console.log(‘Live market update:’, marketData);
// Update the UI with new market data
document.getElementById(‘market-price’).textContent = `Current price: ${marketData.price}`;
};
7. Security Considerations
For production environments, always use secure WebSocket connections (wss://) to ensure data is encrypted. Also, make sure to handle authentication and authorization of users before allowing them to send or receive data.
For example, if your application requires user authentication, you might include a token in the WebSocket URL like this:
const socket = new WebSocket(‘wss://example.com?token=your_auth_token’);
Example of Full WebSocket Integration
Here’s a complete example that shows how to integrate WebSockets into a JavaScript app that handles live market feeds:
const socket = new WebSocket(‘ws://localhost:8080’); // Connect to WebSocket server
// Connection established
socket.onopen = () => {
console.log(‘WebSocket connection established’);
socket.send(‘Requesting market data’);
};
// Handle incoming messages
socket.onmessage = (event) => {
const marketData = JSON.parse(event.data); // Parse the JSON data
console.log(‘Live market update:’, marketData);
// Update the market price on the webpage
document.getElementById(‘market-price’).textContent = `Current price: ${marketData.price}`;
};
// Handle WebSocket errors
socket.onerror = (error) => {
console.error(‘WebSocket error:’, error);
};
// Handle WebSocket closure
socket.onclose = () => {
console.log(‘Connection closed’);
};
How Uses WebSockets with Live Market Feeds
Integrating WebSockets for live market feeds offers a highly efficient way to deliver real-time data to users. Unlike traditional HTTP requests, which require frequent polling to check for updates, WebSockets maintain a persistent connection between the client and the server. This allows for instantaneous transmission of market data, such as stock prices, forex rates, or cryptocurrency values, without the need for repeated requests. As soon as the data changes on the server, it is pushed to the client in real-time, providing a seamless experience for users who rely on up-to-date information to make quick decisions. For example, a WebSocket connection can be established in JavaScript with a simple line of code, enabling the application to receive live updates as they occur, dramatically reducing latency and improving the user experience.
This approach is especially beneficial for financial platforms, where real-time data is critical for activities like trading and decision-making. By leveraging WebSockets, applications can send continuous streams of data, such as the latest stock quotes or cryptocurrency price changes, to users without unnecessary delays. Additionally, WebSockets help reduce server load because data is pushed only when it changes, rather than requiring the server to handle repeated client requests. This efficient method not only enhances performance but also minimizes bandwidth usage, ensuring that live market feeds are delivered with minimal overhead. Ultimately, using WebSockets in live market feeds allows developers to create faster, more responsive applications that meet the demands of real-time data consumption.
Conclusion
Integrating WebSockets in JavaScript for live market feeds provides a robust solution for delivering real-time, low-latency data to users. By maintaining an open, persistent connection between the client and server, WebSockets eliminate the need for frequent polling, allowing for instant updates on market data like stock prices, cryptocurrency values, and forex rates. This not only improves the user experience by providing up-to-the-second information but also reduces server load and bandwidth usage, making it an efficient choice for applications that rely on continuous data streams. Overall, WebSockets enhance the performance and responsiveness of financial platforms and other real-time services, enabling developers to create dynamic, high-performance applications that meet the demands of modern users.